
The original website is long gone, though you can still view it on WayBack. It was adopted by a Scottish musician called Jo Hamilton, who made a pretty cool video with it.
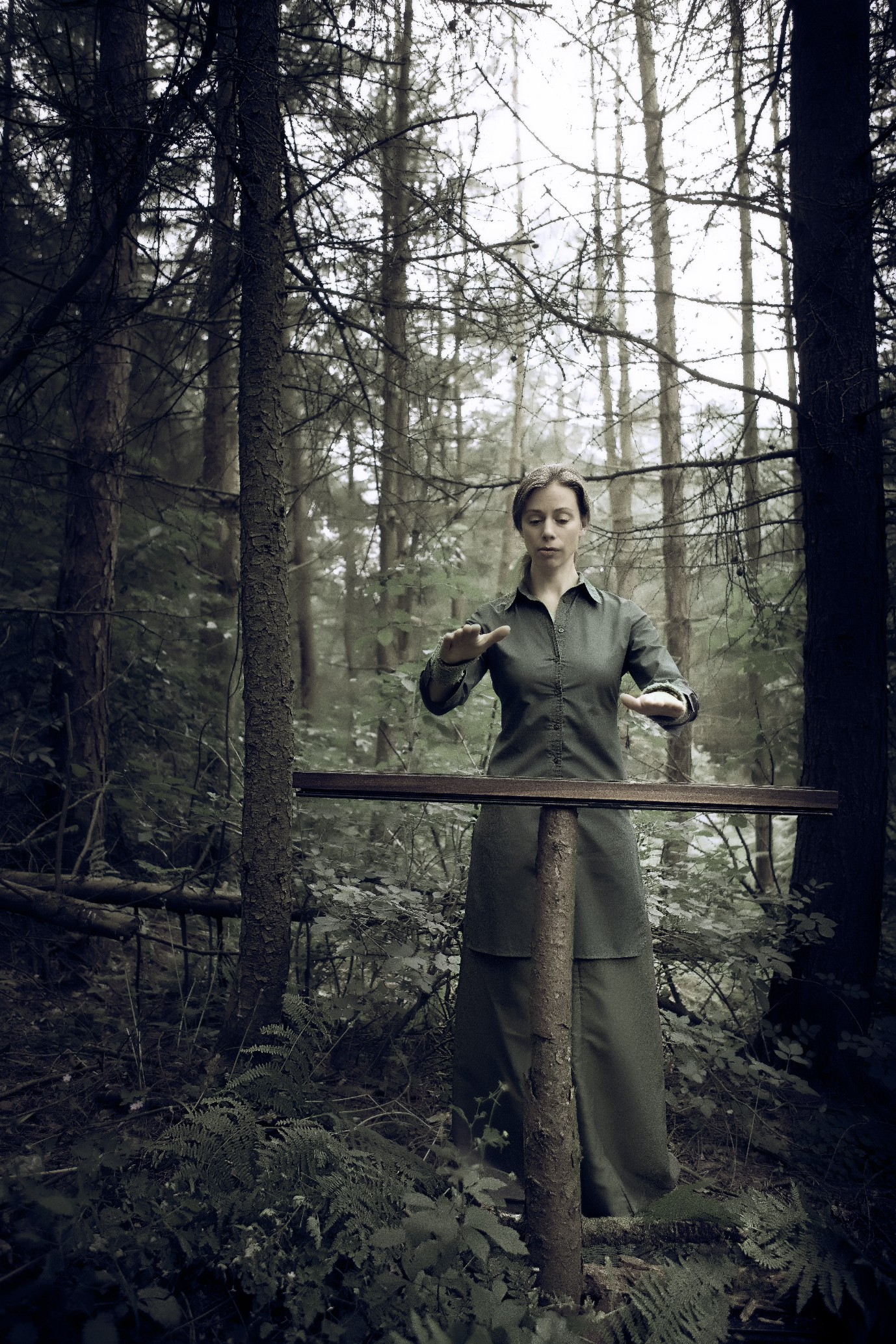
https://youtu.be/DTp5MFgzqZk?t=126
Guardian write-up:
https://www.theguardian.com/music/2011/ ... -air-piano
They were sold in small numbers and then discontinued.
It's based on an Arduino and a set of eight IR sensors. There aren't many instruments around that use IR to trigger notes...the Naonext Crystall Ball (sic) can do it, but it only has five of them, which is a bit limiting. There's also a couple of DIY versions like the Flightdeck, which is conceptually quite close, with eight IR modules.
So inspired by those, I've decided to have a go. I ruthlessly reclaimed the Arduino with its Sparkfun MIDI shield from my last project (byebye Eletrunk), picked up a Sharp GP2Y0A21YK0F on eBay, and I've been messing around with that today.

So far so good...after spending a couple of hours trying to remember how to do some basic coding and sorting out bugs like using "=" instead of "==" I was able to get it to trigger a couple of different MIDI notes for different levels...
https://youtu.be/WVkta4_Yaq8
The original Airpiano seems to have been configured to play a scale, with three octaves for different heights.
Encouraged by this, I just ordered another half dozen sensors, and I think I'll need to upgrade to an Arduino Mega to handle the extra inputs.
My enthusiasm for electronics, programming and woodworking vastly outweighs my skills though sadly, so I'll probably be back asking for help for the next stage. (Need to sort out a decent case next.)